In this guide we will learn how to play with a cheap ‘n’ cheerful temperature and humidity sensor DHT11 – the minuscule 4-pin sensor accurate enough for most do it yourself projects need to read and record humidity and temperature levels.
Sensor Overview
The DHT11 sensor is a perfect blend of one temperature sensor and one humidity sensor that offers calibrated digital signal output through a single-wire serial interface. The sensor in a convenient 4-pin single row pin package holds (as stated above) a resistive-type humidity sensor and an NTC thermistor, and connects to a high-performance 8-bit microcontroller. DHT11 is strictly calibrated in the laboratory and the calibration coefficients are stored as programs in the OTP memory which are used by its internal signal detecting process. The sensor operates on 3-5.5V dc measures temperature in 0-50 degree C (±2) and relative humidity in 20-90% ranges (±5). It can transmit the output signal up to 20-meter thanks to the one-wire, low-power serial communication interface. Take note, out of the 4 pins only 3 pins (1-2-4) are required for playing with the sensor, and a 5K to 10K pull-up resistor is needed in the data line (2) for cable lengths up to 20 meters. Addition of a 100nF capacitor between VDD and GND (1 & 4) will give good power filtering, as well. However, the quality of connection wires will affect the quality and distance of communication, so high-grade shielding-wire is recommended for interconnection.
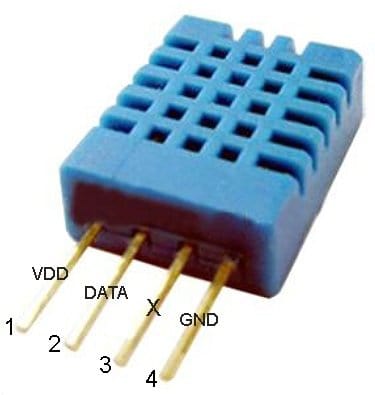
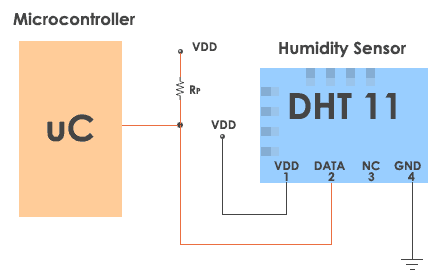
Communication Process
As readers might be noticed, a single-bus data format is used for communication and synchronization between the microcontroller (uC) and DHT11 sensor (one communication process is about 4ms). The data consists of decimal and integral parts, and a complete data transmission is 40bit. The data format is ‘8bit humidity integer data + 8bit the Humidity decimal data +8 bit temperature integer data + 8bit fractional temperature data +8 bit parity bit’ (sensor sends higher data bit first)!
Here’s an example of the data received for 24℃ and 53%RH:
- Data: 0011 0101 (High Humidity 8) 0000 0000 (Low Humidity 8) 0001 1000 (High Temp 8) 0000 0000 (Low Temp 8) 0100 1101 (Parity Bit)
- Checksum: 0011 0101+0000 0000+0001 1000+0000 0000= 0100 1101
- Results: Temperature = 0001 1000=18H=24℃. Humidity = 0011 0101=35H=53%RH.
The Parity bit data definition is “8bit humidity integer data + 8bit humidity decimal data +8 bit temperature integer data + 8bit temperature decimal data”. If the data transmission is right, the check-sum should be the last 8bit (see above – Parity bit is 0100 1101 and the check sum is 0100 1101, yeah).
In the overall communication process, when microcontroller (uC) sends a start signal, the DHT11 sensor wakes up from the low-power-consumption mode to the running-mode, waiting for completing the start signal from the uC. Once it is completed, DHT11 sends a response signal of 40-bit data that include the relative humidity and temperature information to the uC (without the start signal from microcontroller (uC), sensor will not give any response signal). Once data is collected, DHT11 returns to the low power mode until it receives a start signal from the uC again. Shown below is the data timing diagram of the overall communication process.
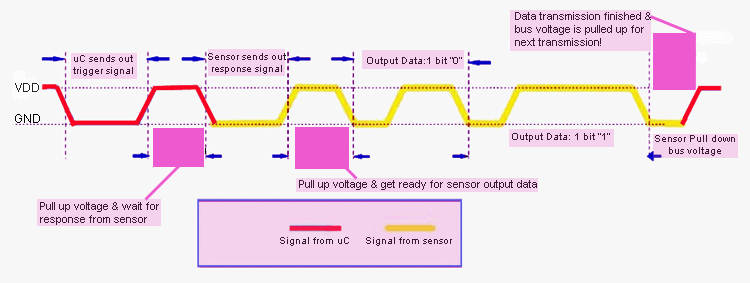
Quick Test
It’d be better for novices to test the functionality of a new DHT11 sensor before rushing into a serious DIY project. Fortunately with an Arduino microcontroller, it’s very easy as there is a good DHT library from Adafruit that will make our task sweet and simple. Once you downloaded the requisite library, just extract it to the Library folder of your Arduino IDE, and copy-paste the simple test code below in your Arduino IDE, compile and upload it to the Arduino board as common. Complex single wire serial protocol of DHT11sensor calls for precise timings indeed but with the mentioned library we don’t have to bother much about the timing patterns as it takes care of all!
// Example test code for DHT11 humidity -temperature sensor // Adapted from the official base code written by Ladyada // Hardware: DHT11 & Arduino Uno R3 //Arduino IDE: 1.6.9 //Prepared & Tested by T.K.Hareendran #include "DHT.h" // DHT Library #define DHTPIN 7 // D7 of Arduino connected with Pin 2 of DHT11 Sensor – 10K Pull Up #define DHTTYPE DHT11 // DHT11 Sensor DHT dht(DHTPIN, DHTTYPE); void setup() { Serial.begin(9600); Serial.println("DHT11 Test »»» "); dht.begin(); } void loop() { delay(2000); float h = dht.readHumidity(); float t = dht.readTemperature(); float f = dht.readTemperature(true); // Check if any reads failed and exit early (to try again). if (isnan(h) || isnan(t) || isnan(f)) { Serial.println("Failed to read from DHT11 sensor!"); return; } // Compute heat index in Fahrenheit (the default) float hif = dht.computeHeatIndex(f, h); // Compute heat index in Celsius (isFahreheit = false) float hic = dht.computeHeatIndex(t, h, false); Serial.print("Humidity: "); Serial.print(h); Serial.print(" %\t"); Serial.print("Temperature: "); Serial.print(t); Serial.print(" *C "); Serial.print(f); Serial.print(" *F\t"); Serial.print("Heat index: "); Serial.print(hic); Serial.print(" *C "); Serial.print(hif); Serial.println(" *F"); }
& Here’s a random capture of author’s serial monitor window
Heat Index?
Keep in mind that Relative Humidity is the ratio of water vapor present in a given volume of air at a given temperature expressed as a percent, and whether we feel warm or cold is determined (among other factors) by the humidity. When humidity (the amount of moisture or water vapor present in the air) is extremely low (i.e. if the air is dry) we may not feel very warm even at moderately high temperature levels, but we feel very embarrassed in the same situation if there is so much moisture in the air.
Yes, the 3rd constituent in the output is Heat Index. The Heat Index is the measure of how hot it really feels when relative humidity is factored with the actual air temperature. For example, if the air temperature is 26°C and the relative humidity is 80%, the estimated heat index (how hot we feel) is 28°C.
The heat index can have many potentially severe medical effects. Sweat is our body’s physiological response to high temperatures, and is an attempt to lower body temperature through evaporation of sweat. When this is hindered, overheating and dehydration can occur, with varying severity. Below is a table indicating possible complications at varying levels of heat index values (Courtesy of the National Weather Service).
Okay, then what about building your own “soapbox” contains a thermometer and hygrometer with a fantastic digital display to notify/update you about the temperature, relative humidity, and heat index levels? I’m in the middle of such a simple yet smart IoT project, and it’s likely to be available to you all before long. Stay tuned…
Disclaimer: Technical details of the DHT11 sensor included here was lifted from the machine-translated version of a Chinese datasheet. The author holds no responsibility for the accuracy of the translated information!